One of Docker's fundamental challenges is managing persistent data. Containers are ephemeral by design—when they're removed, any data stored inside is lost. Docker volumes solve this problem by providing mechanisms to persist and manage data independently of the container lifecycle. In this guide, we'll explore Docker volumes with practical examples and best practices.
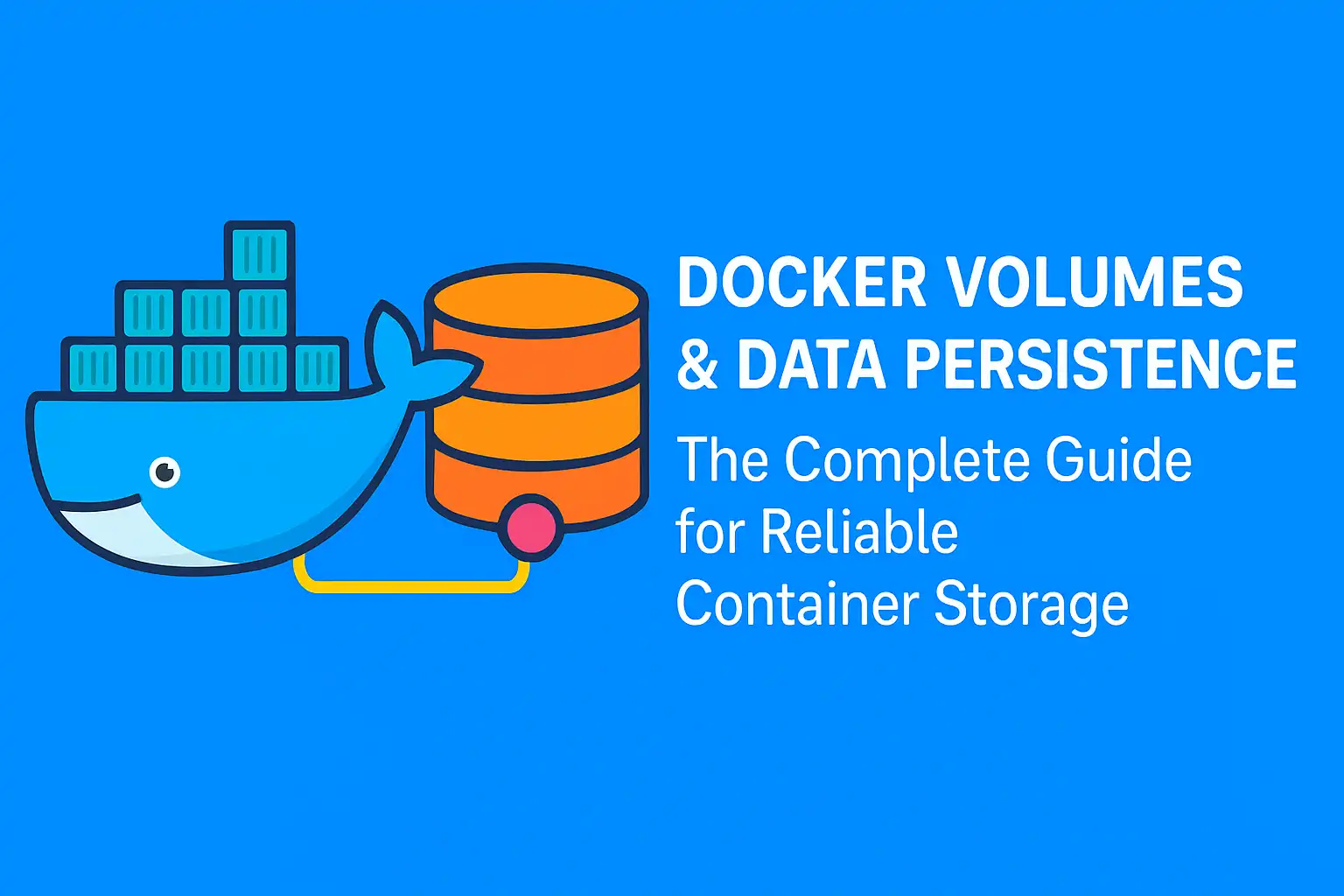
Table of Contents #
- Introduction to Docker Storage
- Understanding Docker's Storage Options
- Docker Volumes in Depth
- Bind Mounts: Connecting to Your Filesystem
- Practical Example: Web Server with Persistent Content
- Volume Management Best Practices
- Working with Docker Compose and Volumes
- Backing Up and Migrating Volume Data
- Common Volume Challenges and Solutions
- Cleanup
- Conclusion
Introduction to Docker Storage #
When working with Docker containers, data persistence is a critical consideration. Docker provides several options for storing data, each with its own use cases and benefits. This guide is based on our Lab2 Persistent Volume Example from the Docker Practical Guide repository.
Understanding Docker's storage options is essential for:
- Persisting application data across container restarts and removals
- Sharing data between containers
- Developing applications with local changes reflected in containers
- Backing up critical data
- Managing stateful applications in containerized environments
Understanding Docker's Storage Options #
Docker provides three primary storage options:
┌─────────────────────────────────────────────────────────────┐
│ Docker Storage Options │
│ │
│ ┌─────────────────┐ ┌─────────────────┐ │
│ │ │ │ │ │
│ │ Container 1 │ │ Container 2 │ │
│ │ │ │ │ │
│ └────────┬────────┘ └────────┬────────┘ │
│ │ │ │
│ │ │ │
│ ▼ ▼ │
│ ┌────────────────┐ ┌────────────────┐ ┌────────────┐ │
│ │ │ │ │ │ │ │
│ │ Docker Volume │ │ Bind Mount │ │ tmpfs │ │
│ │ │ │ │ │ │ │
│ └────────────────┘ └────────────────┘ └────────────┘ │
│ │ │ │
│ ▼ ▼ │
│ ┌────────────────┐ ┌────────────────┐ │
│ │ │ │ │ │
│ │Docker Managed │ │ Host File │ │
│ │Storage │ │ System │ │
│ │ │ │ │ │
│ └────────────────┘ └────────────────┘ │
│ │
└─────────────────────────────────────────────────────────────┘
- Volumes: Docker-managed data storage independent of the container lifecycle
- Bind Mounts: Direct mapping to a host filesystem path
- tmpfs Mounts: Temporary storage in the host system's memory (non-persistent)
Let's explore each option in more detail.
Docker Volumes in Depth #
Volumes are the preferred mechanism for persisting data in Docker. They're completely managed by Docker and stored in a part of the host filesystem that's managed by Docker (/var/lib/docker/volumes/
on Linux).
Key characteristics of Docker volumes:
- Persistence: Data remains even after containers are removed
- Sharing: Multiple containers can mount the same volume
- Safety: Isolated from the host filesystem's core functionality
- Drivers: Support for remote storage, encryption, and other features
- Performance: Often better performance than bind mounts
- Portability: Easier to back up, restore, and migrate
Creating and Managing Volumes #
Let's look at basic volume commands:
# Create a named volume
docker volume create my_data
# List all volumes
docker volume ls
# Inspect a volume
docker volume inspect my_data
# Remove a volume
docker volume rm my_data
# Remove all unused volumes
docker volume prune
Using Volumes with Containers #
Volumes can be attached to containers at runtime:
# Run a container with a volume
docker run -d \
--name nginx_container \
-v my_data:/usr/share/nginx/html \
-p 8080:80 \
nginx
# The same volume can be used by another container
docker run -d \
--name backup_container \
-v my_data:/backup \
alpine \
sleep infinity
Bind Mounts: Connecting to Your Filesystem #
Bind mounts map a specific path on the host to a path in the container. They're excellent for development environments, but less portable than volumes.
Key characteristics of bind mounts:
- Direct Mapping: Maps directly to host filesystem paths
- Host Control: The host manages the storage location and content
- Development: Great for providing source code to containers
- Existing Files: Can access host files directly from containers
- Performance Impact: May have performance constraints on macOS and Windows
- Less Portability: Dependent on the host's filesystem structure
Using Bind Mounts #
# Run a container with a bind mount
docker run -d \
--name web_server \
-v $(pwd)/html:/usr/share/nginx/html \
-p 8080:80 \
nginx
# In the above command, $(pwd)/html on the host is mapped to
# /usr/share/nginx/html in the container
Practical Example: Web Server with Persistent Content #
Let's build a practical example using an Nginx web server with persistent content. This example is based on our Lab2 Persistent Volume Example.
First, let's create a simple HTML file on our host:
mkdir -p html
echo '<h1>Hello from Docker Volume!</h1>' > html/index.html
Next, let's create a Dockerfile for our custom Nginx image:
FROM nginx:alpine
COPY html /usr/share/nginx/html
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
We have two options for running this:
Option 1: Using a Bind Mount for Development #
docker run -d \
--name nginx-dev \
-v $(pwd)/html:/usr/share/nginx/html \
-p 8080:80 \
nginx:alpine
With this approach:
- Files in your
html
directory are directly mapped into the container - Changes to files are immediately reflected in the running container
- When the container is removed, your files remain on your host
Option 2: Using a Named Volume for Production #
# Create a named volume
docker volume create web_content
# Copy initial content to the volume using a temporary container
docker run --rm \
-v $(pwd)/html:/source \
-v web_content:/dest \
alpine \
cp -r /source/* /dest/
# Run the web server with the volume
docker run -d \
--name nginx-prod \
-v web_content:/usr/share/nginx/html \
-p 8080:80 \
nginx:alpine
With this approach:
- Data is managed by Docker in a named volume
- Data persists even if the container is removed
- Multiple containers can use the same volume
- The volume can be backed up, migrated, and managed independently
Testing Our Persistent Volume #
Let's verify that our data persists across container lifecycles:
- Start by accessing the web page at http://localhost:8080 to see our content
- Remove the container:
docker rm -f nginx-prod
- Create a new container using the same volume:
docker run -d \
--name nginx-prod-2 \
-v web_content:/usr/share/nginx/html \
-p 8080:80 \
nginx:alpine - Access the web page again at http://localhost:8080
- The content persists, demonstrating successful data persistence!
Volume Management Best Practices #
To effectively manage Docker volumes in production environments, follow these best practices:
- Use named volumes for production data: Named volumes are easier to manage and back up
- Use bind mounts for development: Bind mounts make it easy to edit code and see changes immediately
- Use read-only volumes where possible: Add
:ro
to volume mounts when containers only need read access - Set proper ownership and permissions: Ensure container processes have appropriate access to the volume
- Regular backups: Backup your volume data regularly
- Clean up unused volumes: Run
docker volume prune
periodically to remove unused volumes - Document volume usage: Keep track of which volumes are used by which applications
- Use volume labels: Add metadata to volumes with labels for better organization
Working with Docker Compose and Volumes #
Docker Compose makes volume management more straightforward with declarative syntax:
version: "3"
services:
webserver:
image: nginx:alpine
volumes:
- web_content:/usr/share/nginx/html
ports:
- "8080:80"
volumes:
web_content:
# By default, this creates a named volume
# Optional: driver: local
This configuration:
- Creates a named volume called
web_content
- Mounts it to
/usr/share/nginx/html
in the container - Persists the data across container restarts and removals
To use this configuration:
docker-compose up -d
To stop containers but keep the volumes:
docker-compose down
To remove containers and volumes:
docker-compose down -v
Backing Up and Migrating Volume Data #
Backing up and migrating Docker volumes is a critical operational task for production systems:
┌─────────────────────────────────────────────────────────────┐
│ Volume Backup Process │
│ │
│ ┌────────────────┐ ┌────────────────┐ │
│ │ │ │ │ │
│ │ Source Volume │ │ Backup │ │
│ │ │ │ Container │ │
│ └───────┬────────┘ └───────┬────────┘ │
│ │ │ │
│ │ │ │
│ ▼ ▼ │
│ ┌──────────────────────────────────────────────┐ │
│ │ │ │
│ │ Mount source volume and backup │ │
│ │ to host or another volume │ │
│ │ │ │
│ └──────────────────────────────────────────────┘ │
│ │
└─────────────────────────────────────────────────────────────┘
Backup a Volume #
# Create a backup of a volume to a tar file
docker run --rm \
-v web_content:/source:ro \
-v $(pwd):/backup \
alpine \
tar -czf /backup/web_content_backup.tar.gz -C /source .
Restore a Volume #
# Restore a backup to a new volume
docker volume create web_content_restored
docker run --rm \
-v web_content_restored:/dest \
-v $(pwd):/backup \
alpine \
sh -c "tar -xzf /backup/web_content_backup.tar.gz -C /dest"
Migrate Volumes Between Hosts #
- Backup the volume on the source host
- Transfer the backup file to the destination host
- Create a new volume on the destination host
- Restore the backup to the new volume
- Update your container configurations to use the new volume
Common Volume Challenges and Solutions #
1. Permission Issues #
Challenge: Container can't write to the volume due to permission issues.
Solution:
# Fix permissions by running a temporary container
docker run --rm \
-v web_content:/data \
alpine \
chown -R 101:101 /data # 101:101 is the nginx user:group in Alpine
2. Volume Not Persisting Data #
Challenge: Data isn't persisting after container removal.
Solutions:
- Ensure you're using a named volume, not an anonymous volume
- Check if you're accidentally removing the volume with
docker-compose down -v
- Verify the volume mount paths are correct
3. Volume Path Conflicts #
Challenge: Multiple containers need different paths to the same volume.
Solution: Use volume mount options to map different container paths:
# Container 1
docker run -d \
-v web_content:/app/data \
my-app-1
# Container 2
docker run -d \
-v web_content:/different/path \
my-app-2
4. Performance Issues #
Challenge: Volume operations are slow, especially on non-Linux hosts.
Solutions:
- For development environments with frequent file changes, consider specific volume types or caching options
- On macOS, use
delegated
orcached
mount options - Consider third-party volume plugins optimized for performance
Cleanup #
When you're done experimenting with Docker volumes, it's important to clean up your environment to free up space and avoid resource conflicts. Here's how to properly clean up volume-related Docker resources:
Stopping and Removing Containers #
First, stop and remove any running containers that are using volumes:
# List running containers
docker ps
# Stop a specific container
docker stop nginx-demo
# Stop all running containers
docker stop $(docker ps -q)
# Remove a specific container
docker rm nginx-demo
# Remove all stopped containers
docker rm $(docker ps -aq)
Managing Volumes #
To list, remove, and manage your volumes:
# List all volumes
docker volume ls
# Inspect a volume to see its details
docker volume inspect web_content
# Remove a specific volume
docker volume rm web_content
# Remove unused volumes (those not attached to containers)
docker volume prune
If you've used Docker Compose, you can clean up everything with:
# Remove containers, networks, and volumes defined in docker-compose.yml
docker-compose down -v
Complete Cleanup #
For a more thorough cleanup of your volume examples:
# Remove all resources related to this tutorial
docker stop nginx-demo nginx-prod nginx-prod-2
docker rm nginx-demo nginx-prod nginx-prod-2
docker volume rm web_content
# Remove backup files if created
rm -f web_content_backup.tar.gz
Verifying Cleanup #
After cleanup, verify that your resources have been removed:
# Check that containers are gone
docker ps -a | grep nginx
# Check that volumes are gone
docker volume ls | grep web_content
This systematic cleanup ensures your system stays clean and ready for your next Docker project, without leftover volumes consuming disk space.
Conclusion #
Docker volumes are essential for managing persistent data in containerized applications. By understanding the differences between volumes and bind mounts, you can choose the right storage option for your specific use case.
In this guide, we've explored:
- Different Docker storage options
- Creating and managing Docker volumes
- Using bind mounts for development
- A practical example with an Nginx web server
- Volume management best practices
- Working with Docker Compose
- Backing up and migrating volume data
- Solving common volume challenges
By implementing these practices, you can ensure that your containerized applications handle data persistence properly, making them more robust and suitable for production environments.
In the next article in our Docker Practical Guide series, we'll dive into Docker networking concepts, exploring how containers communicate with each other and with the outside world. Stay tuned!
How are you handling data persistence in your Docker projects? Share your experiences, challenges, or questions in the comments below!