Effective Docker image management is crucial for maintaining a streamlined, secure, and efficient containerized workflow. From tagging strategies to security considerations, proper image management practices help teams collaborate more effectively and deploy with confidence. In this guide, we'll explore comprehensive best practices for managing Docker images throughout their lifecycle.
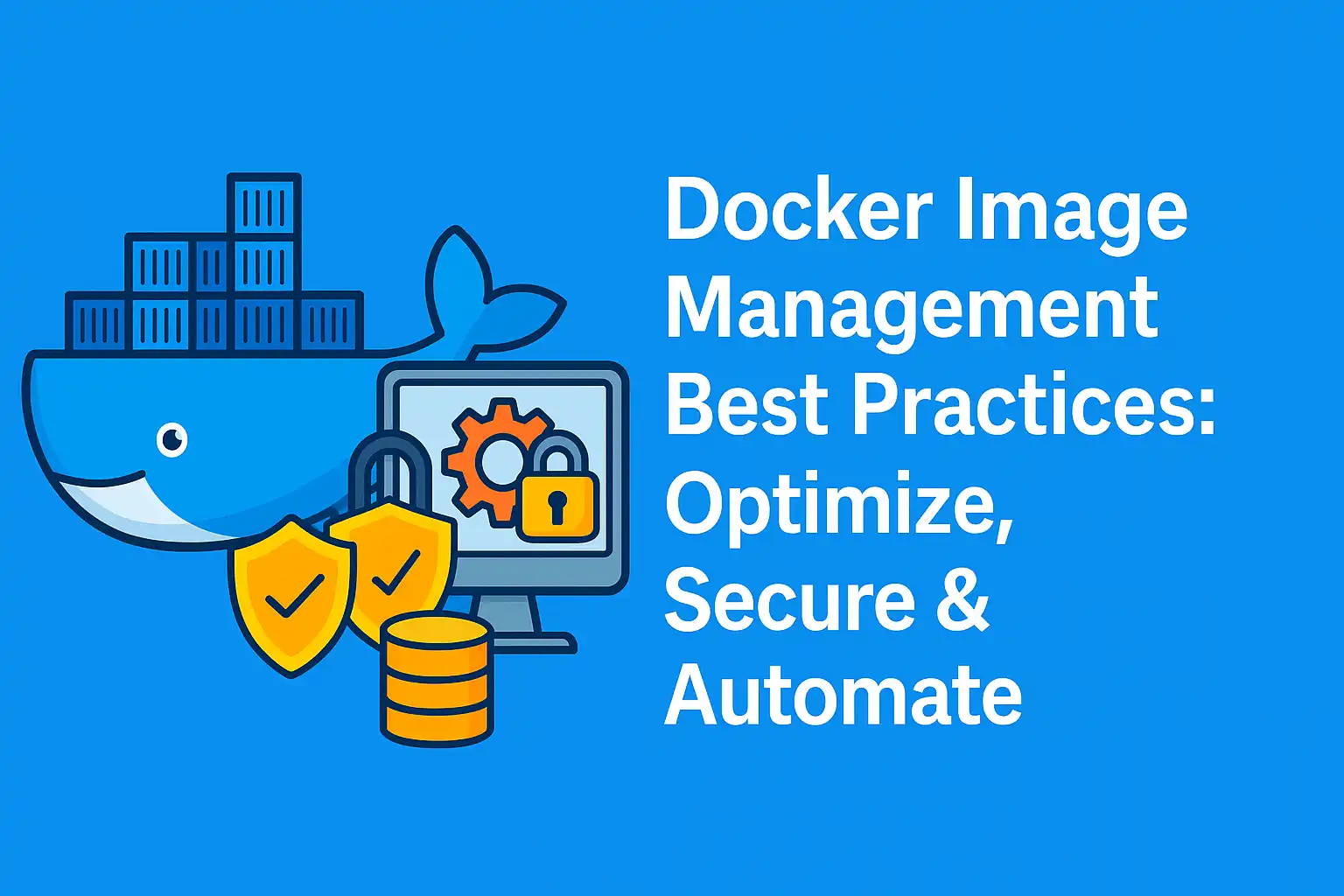
Go To...
- Introduction to Docker Image Management
- Building Effective Docker Images
- Image Tagging Strategies
- Working with Docker Registries
- Security Best Practices
- Docker Image Optimization
- Image Distribution Techniques
- Automating Image Management
- Pruning and Cleanup
- Practical Examples
- Conclusion
Introduction to Docker Image Management
Docker images are the building blocks of containers, containing everything needed to run an application: code, runtime, libraries, and system tools. This guide is based on our Lab5 Image Management Example from the Docker Practical Guide repository.
Effective image management addresses several critical aspects:
- Consistency: Ensuring consistent environments across development, testing, and production
- Versioning: Tracking changes and enabling rollbacks
- Distribution: Efficiently sharing images across teams and environments
- Security: Protecting images from vulnerabilities and unauthorized access
- Storage Optimization: Minimizing storage requirements and transfer times
Let's explore these aspects in detail with practical examples.
Building Effective Docker Images
Before diving into management strategies, it's important to understand different approaches to building Docker images:
1. Basic Dockerfile
The simplest approach uses a single-stage build:
FROM python:3.9
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
2. Multi-Stage Builds
For optimized images, use multi-stage builds to separate build and runtime environments:
# Build stage
FROM python:3.9 AS builder
WORKDIR /app
COPY requirements.txt .
RUN pip install --user -r requirements.txt
# Run stage
FROM python:3.9-slim
WORKDIR /app
COPY /root/.local /root/.local
COPY . .
ENV PATH=/root/.local/bin:$PATH
CMD ["python", "app.py"]
3. Non-Root User Images
For enhanced security, run containers as non-root users:
FROM python:3.9-slim
# Create non-root user
RUN useradd -m appuser
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
# Switch to non-root user
USER appuser
CMD ["python", "app.py"]
Image Tagging Strategies
Proper tagging is essential for managing Docker images effectively:
┌───────────────────────────────────────────────────────────┐
│ Docker Image Tagging │
│ │
│ ┌─────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Image: myapp │ │
│ │ │ │
│ │ Tags: │ │
│ │ ├── latest │ │
│ │ ├── 1.0.0 │ │
│ │ ├── 1.0 │ │
│ │ ├── stable │ │
│ │ ├── v1.0.0-alpine │ │
│ │ ├── dev │ │
│ │ ├── 20240612 │ │
│ │ └── commit-a1b2c3d │ │
│ │ │ │
│ └─────────────────────────────────────────────────────┘ │
│ │
└───────────────────────────────────────────────────────────┘
Semantic Versioning
Use semantic versioning (SemVer) for production images:
# Build with semantic version tags
docker build -t myapp:1.2.3 .
# Add additional tags
docker tag myapp:1.2.3 myapp:1.2
docker tag myapp:1.2.3 myapp:1
docker tag myapp:1.2.3 myapp:latest
Environment-Based Tagging
Use tags to indicate the target environment:
docker build -t myapp:dev .
docker build -t myapp:staging .
docker build -t myapp:production .
Date-Based Tagging
Include dates for better traceability:
# Format: YYYYMMDD
docker build -t myapp:20240612 .
# Combine with version
docker build -t myapp:1.2.3-20240612 .
Git Commit-Based Tagging
Connect images to their source code:
# Use git commit hash in tag
COMMIT_HASH=$(git rev-parse --short HEAD)
docker build -t myapp:commit-${COMMIT_HASH} .
Working with Docker Registries
Docker registries store and distribute your images. Here's how to work with them effectively:
Docker Hub
Docker Hub is the default public registry:
# Log in to Docker Hub
docker login
# Push an image
docker push username/myapp:1.0.0
# Pull an image
docker pull username/myapp:1.0.0
Private Registries
For proprietary images, use private registries:
# Log in to private registry
docker login registry.example.com
# Tag for private registry
docker tag myapp:1.0.0 registry.example.com/team/myapp:1.0.0
# Push to private registry
docker push registry.example.com/team/myapp:1.0.0
Working with Registry Credentials
Manage credentials securely:
# Store credentials in config.json
docker login --username myuser --password-stdin < password.txt
# Use credential helpers
docker login --username myuser --password-stdin registry.example.com
Security Best Practices
Security is paramount when managing Docker images:
1. Scan Images for Vulnerabilities
Regularly scan your images for known vulnerabilities:
# Using Docker Scout (formerly Docker Scan)
docker scout cves myapp:1.0.0
# Using Trivy (popular open-source scanner)
trivy image myapp:1.0.0
2. Use Minimal Base Images
Reduce attack surface with minimal base images:
# Instead of full Ubuntu
FROM ubuntu:20.04
# Use smaller Alpine
FROM alpine:3.15
# Or distroless for even smaller images
FROM gcr.io/distroless/static-debian11
3. Run as Non-Root User
Prevent privilege escalation by running as non-root:
# Create non-root user
RUN useradd -m appuser
# Set ownership
COPY . .
# Switch to non-root user
USER appuser
4. Use Multi-Stage Builds
Separate build-time dependencies from runtime:
FROM node:16 AS build
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
RUN npm run build
FROM node:16-alpine
WORKDIR /app
COPY /app/dist ./dist
USER node
CMD ["node", "dist/index.js"]
5. Sign and Verify Images
Ensure image authenticity with signing:
# Sign an image
docker trust sign username/myapp:1.0.0
# Enable content trust
export DOCKER_CONTENT_TRUST=1
# Pull only signed images
docker pull username/myapp:1.0.0
Docker Image Optimization
Optimized images improve performance and resource usage:
1. Minimize Layers
Combine related commands to reduce layers:
# Instead of multiple RUN commands
RUN apt-get update
RUN apt-get install -y package1
RUN apt-get install -y package2
# Combine them
RUN apt-get update && \
apt-get install -y \
package1 \
package2 \
&& rm -rf /var/lib/apt/lists/*
2. Use .dockerignore
Exclude unnecessary files:
# .dockerignore
.git
node_modules
*.log
Dockerfile
.dockerignore
tests
docs
3. Clean Up in the Same Layer
Remove temporary files in the same layer they're created:
RUN apt-get update && \
apt-get install -y some-package && \
apt-get clean && \
rm -rf /var/lib/apt/lists/*
4. Use Specific Versions
Pin dependencies to specific versions:
# Instead of
FROM python:3
# Use specific version
FROM python:3.9.10-slim
Image Distribution Techniques
Efficiently distribute Docker images across environments:
1. Save and Load Images
Transfer images without a registry:
# Save image to a tar file
docker save -o myapp.tar myapp:1.0.0
# Load image from tar file
docker load -i myapp.tar
2. Export and Import Containers
Capture a container's filesystem:
# Create a container
docker create --name mycontainer myapp:1.0.0
# Export container filesystem
docker export mycontainer > myapp-container.tar
# Import as a new image
cat myapp-container.tar | docker import - myapp:imported
3. Image Layers Caching
Leverage build caching for faster builds:
# Copy files that change less frequently first
COPY package.json .
RUN npm install
# Then copy application code that changes more often
COPY . .
Automating Image Management
Automate image management tasks with CI/CD:
GitHub Actions Example
name: Docker Build and Push
on:
push:
branches: [main]
tags: ["v*"]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v2
- name: Login to DockerHub
uses: docker/login-action@v2
with:
username: $
password: $
- name: Extract metadata
id: meta
uses: docker/metadata-action@v4
with:
images: username/myapp
tags: |
type=semver,pattern=
type=semver,pattern=.
type=semver,pattern=
type=ref,event=branch
- name: Build and push
uses: docker/build-push-action@v3
with:
context: .
push: true
tags: $
labels: $
Pruning and Cleanup
Maintain a clean Docker environment:
# Remove unused images
docker image prune
# Remove all unused images (not just dangling ones)
docker image prune -a
# Remove unused containers, networks, and images
docker system prune
# Remove everything unused including volumes
docker system prune -a --volumes
Set up automated cleanup schedules:
# Cron job to clean up dangling images weekly
0 0 * * 0 docker image prune -f > /dev/null 2>&1
Practical Examples
Let's implement some practical image management examples based on our Lab5:
Example 1: Build and Tag Multiple Variants
#!/bin/bash
# Build base image
docker build -t myapp:base -f Dockerfile.basic .
# Build multi-stage optimized image
docker build -t myapp:optimized -f Dockerfile.multistage .
# Build secure non-root image
docker build -t myapp:secure -f Dockerfile.nonroot .
# Tag with date
DATE=$(date +%Y%m%d)
docker tag myapp:secure myapp:secure-${DATE}
# Tag for registry
docker tag myapp:secure registry.example.com/myteam/myapp:secure
Example 2: Image Size Comparison
#!/bin/bash
echo "Image size comparison:"
echo "---------------------"
docker images --format ": - " | grep myapp
Output:
myapp:base - 945MB
myapp:optimized - 125MB
myapp:secure - 128MB
Example 3: Save and Distribute Images
#!/bin/bash
# Create directory for saved images
mkdir -p images
# Save optimized image
docker save myapp:optimized -o images/myapp-optimized.tar
# Compress the image for distribution
gzip images/myapp-optimized.tar
echo "Image saved to images/myapp-optimized.tar.gz"
echo "File size: $(du -h images/myapp-optimized.tar.gz | cut -f1)"
Example 4: Scan for Vulnerabilities
#!/bin/bash
# Scan the secure image
docker scout cves myapp:secure
# Generate HTML report
docker scout cves --format html --output security-report.html myapp:secure
echo "Security scan complete. Report saved to security-report.html"
Cleanup
Managing Docker images requires regular cleanup to prevent disk space issues and maintain system performance. Since Docker images can consume significant storage, especially when working with multiple variants and versions, a comprehensive cleanup strategy is essential.
Identifying Space Usage
Before cleaning up, identify what's consuming space:
# Check disk usage by Docker
docker system df
# Get detailed image size information
docker system df -v
# List all images with sizes
docker images --format ": - "
# Sort images by size (requires jq)
docker images --format '\t:' | sort -hr
Cleaning Up Images
Remove unused images in several ways:
# Remove a specific image
docker rmi myapp:old-version
# Remove multiple images
docker rmi myapp:v1.0.0 myapp:v1.1.0
# Remove images by ID
docker rmi 3f4ab8d12fe0 946c0c620b12
# Remove dangling images (untagged images)
docker image prune
# Remove all unused images (not just dangling)
docker image prune -a
# Remove images with a specific filter
docker image prune --filter "until=24h"
Registry Cleanup
Clean up images from registries:
# Remove local images after pushing to registry
docker push myregistry.com/myapp:latest && docker rmi myapp:latest
# Use skopeo to remove registry images without pulling
skopeo delete docker://myregistry.com/myapp:old-tag
Docker Build Cache Cleanup
Multi-stage builds and frequent rebuilds create build cache:
# Remove build cache
docker builder prune
# Remove all builder cache
docker builder prune --all
# Set build cache size limit in daemon.json
# { "builder": { "gc": { "enabled": true, "defaultKeepStorage": "10GB" } } }
Lab Cleanup Commands
For the specific image management examples in our Lab5:
# Navigate to the lab directory
cd lab5_image_management_example
# Run the cleanup script
./cleanup.sh
# Or manually clean up
docker rm -f $(docker ps -aq --filter name=myapp)
docker rmi myapp:base myapp:optimized myapp:secure
docker rmi $(docker images -q registry.example.com/myteam/myapp)
# Clean up saved image files
rm -rf images/
Establishing a regular cleanup routine as part of your image management workflow prevents system degradation and ensures optimal performance for your Docker environment.
Conclusion
Effective Docker image management is a cornerstone of successful containerization strategies. By implementing proper tagging, security measures, optimization techniques, and automation, you can streamline your container workflows and ensure consistent, reliable deployments.
In this guide, we've explored:
- Different approaches to building Docker images
- Strategic image tagging conventions
- Working with Docker registries
- Security best practices for image management
- Optimization techniques for smaller, faster images
- Efficient image distribution methods
- Automation of image management tasks
- Cleanup strategies to maintain a healthy Docker environment
With these practices in place, your team can collaborate more effectively, deploy with confidence, and maintain a secure and efficient container ecosystem.
In the next article in our Docker Practical Guide series, we'll explore Docker Swarm for container orchestration across multiple hosts. Stay tuned!
What image management challenges have you faced in your Docker workflows? Share your experiences or questions in the comments below!
Join the Discussion
Share your thoughts and insights below
Ready to Comment?
Click the button below to load the discussion and share your thoughts with the community.
Loading Comments...
Please wait while we load the discussion.